2020. 5. 14. 11:14ㆍ프로그래밍언어/Java&Servlet
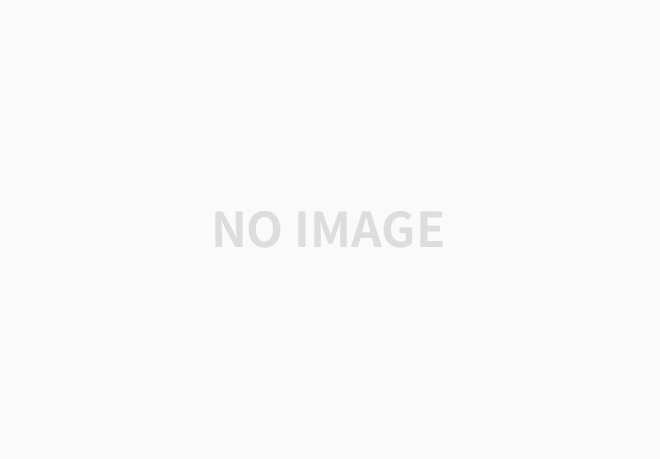
오늘 다루어볼 내용은 java 14에서 도입된 record 타입의 클래스입니다.
record란?
레코드(record)란 "데이터 클래스"이며 순수하게 데이터를 보유하기 위한 특수한 종류의 클래스이다. 코틀린의 데이터 클래스와 비슷한 느낌이라고 보면 된다. 밑에서 코드를 보겠지만, record 클래스를 정의할때, 그 모양은 정말 데이터의 유형만 딱 나타내는 듯한 느낌이다. 훨씬더 간결하고 가볍기 때문에 Entity 혹은 DTO 클래스를 생성할때 사용되면 굉장히 좋을 듯하다.
sample code
간단하게 샘플코드를 살펴보자.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
public class SampleRecord {
private final String name;
private final Integer age;
private final Address address;
public SampleRecord(String name, Integer age, Address address) {
this.name = name;
this.age = age;
this.address = address;
}
public String getName() {
return name;
}
public Integer getAge() {
return age;
}
public Address getAddress() {
return address;
}
}
|
cs |
위와 같은 코드가 있다고 가정하자. 해당 클래스는 모든 인스턴스 필드를 초기화하는 생성자가 있고, 모든 필드는 final로 정의되어 있다. 그리고 각각 필드의 getter를 가지고 있다. 이러한 클래스 같은 경우는 record 타입의 클래스로 변경이 가능하다.
1
2
3
4
5
|
public record SampleRecord(
String name,
Integer age,
Address address
) {}
|
cs |
엄청 클래스가 간결해진 것을 볼 수 있다. 이 record 클래스에 대해 간단히 설명하면 아래와 같다.
- 해당 record 클래스는 final 클래스이라 상속할 수 없다.
- 각 필드는 private final 필드로 정의된다.
- 모든 필드를 초기화하는 RequiredAllArgument 생성자가 생성된다.
- 각 필드의 getter는 getXXX()가 아닌, 필드명을 딴 getter가 생성된다.(name(), age(), address())
만약 그런데 json serialize가 되기 위해서는 위와 같이 선언하면 안된다. 아래와 같이 jackson 어노테이션을 붙여줘야한다.
1
2
3
4
5
|
public record SampleRecord(
@JsonProperty("name") String name,
@JsonProperty("age") Integer age,
@JsonProperty("address") Address address
) {}
|
cs |
record 클래스는 static 변수를 가질 수 있고, static&public method를 가질 수 있다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
public record SampleRecord(
@JsonProperty("name") String name,
@JsonProperty("age") Integer age,
@JsonProperty("address") Address address
) {
static String STATIC_VARIABLE = "static variable";
@JsonIgnore
public String getInfo() {
return this.name + " " + this.age;
}
public static String get() {
return STATIC_VARIABLE;
}
}
|
cs |
또한 record 클래스의 생성자를 명시적으로 만들어서 생성자 매개변수의 validation 로직등을 넣을 수도 있다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
|
public record SampleRecord(
@JsonProperty("name") String name,
@JsonProperty("age") Integer age,
@JsonProperty("address") Address address
) {
public SampleRecord {
if (name == null || age == null || address == null) {
throw new IllegalArgumentException();
}
}
static String STATIC_VARIABLE = "static variable";
@JsonIgnore
public String getInfo() {
return this.name + " " + this.age;
}
public static String get() {
return STATIC_VARIABLE;
}
}
|
cs |
이러한 record 클래스를 spring의 controller와 연계해서 사용하면 더 간결한 injection이 가능해지기 때문에 훨씬 깔끔한 컨트롤러 클래스작성이 가능하다.
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
|
======================================================================================
public record SampleRecord(
@JsonProperty("name") String name,
@JsonProperty("age") Integer age,
@JsonProperty("address") Address address
) {
static String STATIC_VARIABLE = "static variable";
@JsonIgnore
public String getInfo() {
return this.name + " " + this.age;
}
public static String get() {
return STATIC_VARIABLE;
}
}
======================================================================================
public record Address(
@JsonProperty("si") String si,
@JsonProperty("gu") String gu,
@JsonProperty("dong") String dong
) {}
======================================================================================
@Service
public class SampleRecordService {
public Mono<SampleRecord> sampleRecordMono(SampleRecord sampleRecord) {
return Mono.just(sampleRecord);
}
}
======================================================================================
@RestController
public record SampleController(SampleRecordService sampleRecordService) {
@PostMapping("/")
public Mono<SampleRecord> sampleRecord(@RequestBody SampleRecord sampleRecord) {
System.out.println(sampleRecord.getInfo());
return sampleRecordService.sampleRecordMono(sampleRecord);
}
}
======================================================================================
|
cs |
여기까지 간단하게 jdk 14의 new feature인 record 클래스에 대해 간단하게 다루어 보았다. 모든 코드는 아래 깃헙을 참고하자.
yoonyeoseong/jdk_14_record_sample
Contribute to yoonyeoseong/jdk_14_record_sample development by creating an account on GitHub.
github.com
참조 : https://dzone.com/articles/jdk-14-records-for-spring-devs
JDK 14 Records for Spring - DZone Java
In this article, we'll discuss several use cases for JDK 14 Records to write cleaner and more efficient code.
dzone.com
'프로그래밍언어 > Java&Servlet' 카테고리의 다른 글
Java - Reactor switchIfEmpty 사용시 주의점(Lambda, 람다 Lazy Evaluation) (2) | 2020.07.29 |
---|---|
Java - 자바 클로져(Closure) & 커링(currying) (0) | 2020.07.22 |
Java - Model(Object) mapping을 위한 Mapstruct (맵스트럭트)! (0) | 2020.04.29 |
Java - 중첩이 많은 Stream 처리 Tip ! (0) | 2020.04.21 |
Java - CopyOnWriteArraySet 클래스 (0) | 2019.12.19 |